So, you’re trying to figure out how to print the pascal triangle in Java? Well, you’re in luck! I have the code for you right here. I have also included comments to walk you through how the code is working.
How To Print The Pascal Triangle In Java: Code
import java.util.Scanner;
public class PascalTriangle {
public static void main(String[] args) {
// 1. create a scanner to take user input
Scanner scanner = new Scanner(System.in);
// 2. ask the user to enter the total number of rows in the triangle
System.out.println("Enter the number of row in your pascal triangle: ");
// 3. declare the total triangle rows, spaces and initial number
int triangleRows = scanner.nextInt();
int spaces = triangleRows;
int number = 1;
// 4. Loop through the total number of triangle rows
for(int i=0; i<triangleRows; i++) {
// 5. print a " " for each number of spaces
for(int j=0; j<spaces; j++) {
System.out.print(" ");
}
// 6. reset number variable
number = 1;
// 7. print each number in the current row
for(int k=0; k<=i; k++) {
System.out.print(number + " ");
number = number * (i-k)/(k+1);
}
// 8. reduce the number of spaces by 1 and begin again on a new line
spaces = spaces - 1;
System.out.println();
}
}
}
Output:
Enter the number of row in your pascal triangle: *5*
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
How To Print Pascal’s Triangle In Java: Step-By-Step Algorithm
In order to understand how to print pascal’s triangle in Java, you should know what the Pascal triangle is. You can’t solve an algorithm without understanding what it does!
If you understand what pascal’s triangle is, feel free to skip to the steps below. Pascal’s Triangle is essentially a pyramid of numbers. Each number is the sum of the two parents directly above the current number. If there is no number above the current number, you can assume the number is 0. The pyramid starts at one.
Here is an animated gif to help you understand how the pyramid is built.
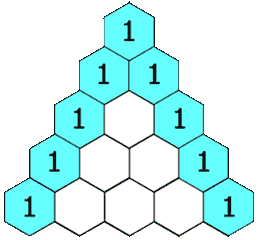
Although creating Pascal’s triangle is relatively simple to do, the triangle is actually a mathematical treasure trove. The scope of mathematical patterns and secrets that are held within Pascal’s triangle is beyond the scope of this text. I highly recommend checking out this video to learn more.
For now, how the triangle is created is enough to understand and implement the algorithm.
Algorithm
- Import the java.util.Scanner object in order to get users input for how many rows are in the triangle
- Create a class called PascalsTriangle that we will write our code in
- Then create a main method within our PascalsTriangle class
- Create a scanner variable to gain user input
- Print a line asking the user to state how many rows the triangle should have
- Create a variable for the triangle rows and set it to user input
- Then create a variable for the number of spaces needed and set it to the triangle rows
- Create a variables for the current number and set it to 1
- Create a loop that will iterate for the total number of rows
- Within the loop created in step 9, Create an inner loop that will create a space for each number in the space variable
- Next, still within the loop created in step 9, Reset the number variable to 1
- Still within the loop created in step 9, create a second inner loop that prints out the number and a space
- Again, within the loop created in step 9, update the number with the formula, number * (current row – current inner loop iteration) / (current inner loop iteration + 1)
- Within the loop created in step 9, reduce the space variable by 1
- Within the loop created in step 9, print a new line
Wrapping Up
That’s pretty much it! That is how to print pascal’s triangle in Java. Pascal’s triangle is a really cool concept in mathematics and I am happy you decided to learn how to implement it in code!
If you are new to programming and want to learn web development, check out my guide here.
If you’re studying for your coding interviews, check out the top languages to study with here.
Happy coding!