Are you having trouble distinguishing the difference between high level and low level programming languages? There’s a good reason for that.
Most people talk about high level and low level programming languages as if they are characteristics of languages themselves. However, that is not the case.
Low level and high level languages exist on a scale, not as a definitive characteristic of a language.
That’s why some people may call C a low level language and others call it a high level language. It really depends on the context that the language is being discussed.
For instance, in contrast to Python, C is a low-level language. However, in comparison to Assembly, C is a high level language.
I know this might be a bit confusing.
So in this article, we are going to discuss what exactly a high level and a low level language is. Then show some examples and diagrams as to why some languages are obviously high or low level languages and why some are considered more ambiguous depending on the context.
What Is A High Level Language And A Low Level Programming Language?
Let’s begin by defining exactly what a low level programming language is. According to Wikipedia, a low-level programming language is one that provides little to no abstraction. In more general terms, it is a language that is easily interpreted by the computer, not the programmer.
We will talk more about abstraction below. As it is an important concept in understanding the difference between high level and low level programming languages.
In contrast to low level language, a high level language is one that has been heavily abstracted. The language caters more to readability and allows the programmer to more easily understand what the code is doing.
That’s pretty much it. More simply put, low level languages are written with the computer in mind, while high level languages are written with the programmer in mind. In order to write large and meaningful programs, we need to utilize both!
Understanding Abstraction
So this brings us to the point of this article. Understanding the difference between high level and low level programming languages begins with abstraction.
Abstraction in programming is the process of hiding away the code that is irrelevant and only providing the code that is necessary. When you write code in any programming language, you are actually writing highly abstracted machine code, or binary code.
If you were to write code in C, here are the levels of abstraction you would see.
Binary Code -> Machine Code -> Assembly Code -> C
Here is a slightly more complex example of the full picture to help you get the idea.
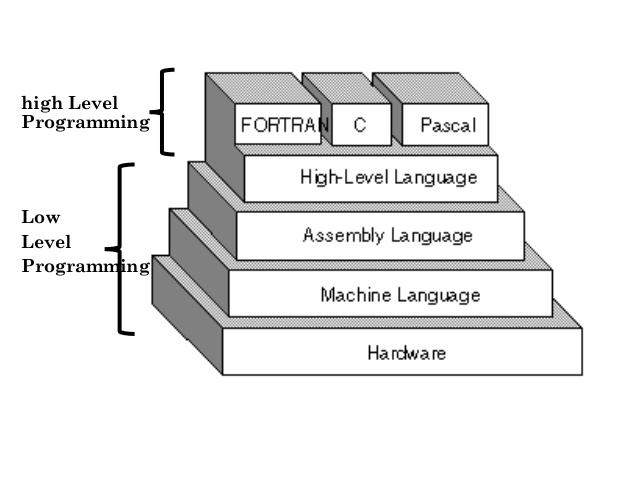
As you can see in this example, C, along with FORTRAN and Pascal are considered high level programming languages.
However, did you know that languages like Python, C#, Java, JavaScript, and PHP are built on top of C? In which case, they are considered higher level languages than C. When people consider C a low or mid level language, they are usually referring to a diagram like this.
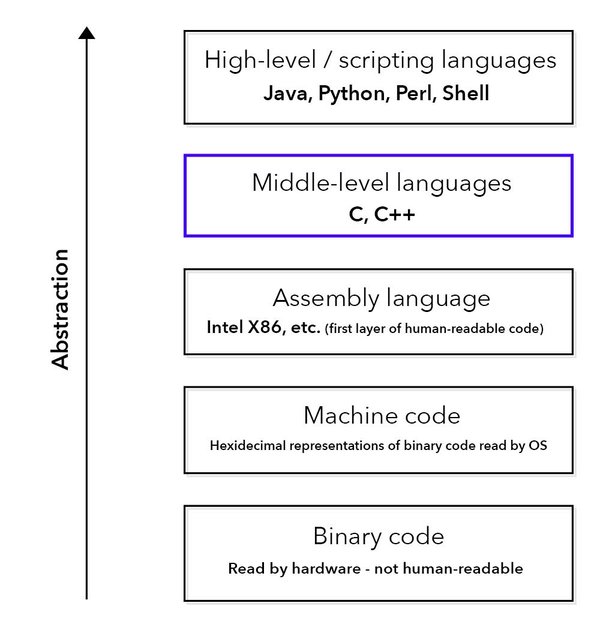
Abstraction Through Example
Abstraction is a pretty cool concept! I thought it would be even cooler to show you how abstraction works through an example.
Below I am going to write “Hello World” in machine code, assembly code, C, and then Python. All of these programs essentially do the same thing, just at lower to higher abstractions.
Let’s dive in!
- “Hello, world!” in Machine Code (32-bit Linux)
00000000 7f 45 4c 46 01 01 01 00 00 00 00 00 00 00 00 00 |.ELF............|
00000010 02 00 03 00 01 00 00 00 80 80 04 08 34 00 00 00 |............4...|
00000020 c8 00 00 00 00 00 00 00 34 00 20 00 02 00 28 00 |........4. ...(.|
00000030 04 00 03 00 01 00 00 00 00 00 00 00 00 80 04 08 |................|
00000040 00 80 04 08 9d 00 00 00 9d 00 00 00 05 00 00 00 |................|
00000050 00 10 00 00 01 00 00 00 a0 00 00 00 a0 90 04 08 |................|
00000060 a0 90 04 08 0e 00 00 00 0e 00 00 00 06 00 00 00 |................|
00000070 00 10 00 00 00 00 00 00 00 00 00 00 00 00 00 00 |................|
00000080 ba 0e 00 00 00 b9 a0 90 04 08 bb 01 00 00 00 b8 |................|
00000090 04 00 00 00 cd 80 b8 01 00 00 00 cd 80 00 00 00 |................|
000000a0 48 65 6c 6c 6f 2c 20 77 6f 72 6c 64 21 0a 00 2e |Hello, world!...|
000000b0 73 68 73 74 72 74 61 62 00 2e 74 65 78 74 00 2e |shstrtab..text..|
000000c0 64 61 74 61 00 00 00 00 00 00 00 00 00 00 00 00 |data............|
000000d0 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 00 |................|
*
000000f0 0b 00 00 00 01 00 00 00 06 00 00 00 80 80 04 08 |................|
00000100 80 00 00 00 1d 00 00 00 00 00 00 00 00 00 00 00 |................|
00000110 10 00 00 00 00 00 00 00 11 00 00 00 01 00 00 00 |................|
00000120 03 00 00 00 a0 90 04 08 a0 00 00 00 0e 00 00 00 |................|
00000130 00 00 00 00 00 00 00 00 04 00 00 00 00 00 00 00 |................|
00000140 01 00 00 00 03 00 00 00 00 00 00 00 00 00 00 00 |................|
00000150 ae 00 00 00 17 00 00 00 00 00 00 00 00 00 00 00 |................|
00000160 01 00 00 00 00 00 00 00 |........|
2. “Hello, world!” in Assembly Code (Linux)
section .text
global _start ;must be declared for linker (ld)
_start: ;tell linker entry point
mov edx,len ;message length
mov ecx,msg ;message to write
mov ebx,1 ;file descriptor (stdout)
mov eax,4 ;system call number (sys_write)
int 0x80 ;call kernel
mov eax,1 ;system call number (sys_exit)
int 0x80 ;call kernel
section .data
msg db 'Hello, world!',0xa ;our dear string
len equ $ - msg ;length of our dear string
3. “Hello, world!” in C
#include <stdio.h>
int main() {
printf("Hello, world!");
return 0;
}
4. “Hello, world!” in Python
print("Hello, world")
Hopefully this was obvious, but did you see how each iteration was considerably easier to read and write? That’s the power of abstraction!
Summary
So that’s pretty much it! That’s the difference between high level and low level programming languages.
To summarize, high level programming languages are languages that are easier for humans to interpret. While low level languages are easier for machines to interpret. High and low level languages work together to create robust and sophisticated software through the process of abstraction.
Looking for more to read? Check out my article on 7 types of algorithms every developer should know and 8 steps to becoming a web developer without a degree.
If you are a new developer looking to learn web development, check out my list of free resources here.
Happy coding!