So you want to know how to undo the most recent local commits in git? As programmers, sometimes we make mistakes. The good news is that Git makes it easy to retract these recent commits and get you back to your previous version of your codebase quickly.
Let’s not waste any time and jump right in!
Undo Local Commit [Quick Summary]
In case you are like most programmers and blindly copy and paste things from the internet without ever reading them, here are all the commands to use in order. These commands will work whether you are using Windows, macOS, or Linux.
:: use this command to find the hash of the commit you need
> git log --oneline
:: use this command to revert this commit
> git revert [hash] --no-edit
Undo Local Commit [Example + Screenshots]
Original State of Project
So if the above example wasn’t clear enough, I am going to walk you through the above steps with a few screenshots and in-depth explanations.
First, let’s start with a blank folder. Make sure that git is installed!
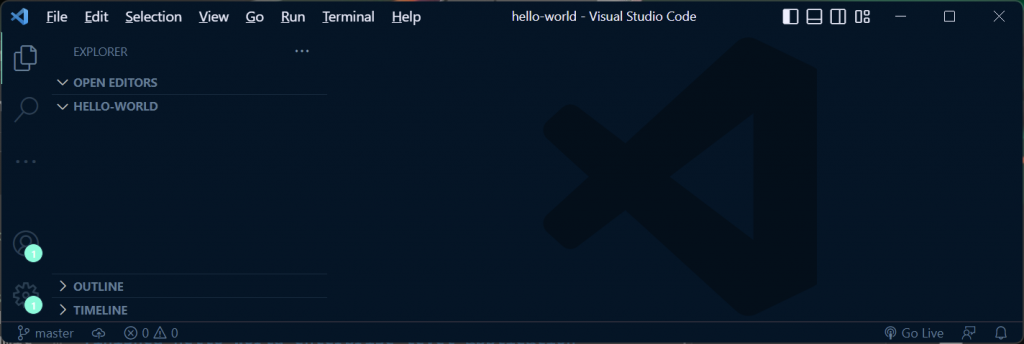
Add Code To Our Project
Now let’s say we want to write an enterprise-level JavaScript application to say ‘hello world’ so that we can become millionaires and quit our jobs.
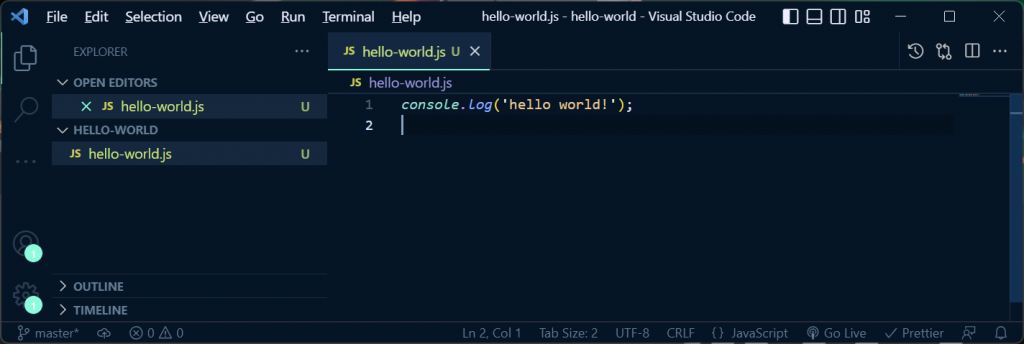
Check, Add, and Commit Our Changes
Now, let’s go over to our PowerShell / Terminal / GitBash / wherever you are running your commands.
The first thing we are going to run is:
> git status
This will show us there are no commits yet but we have an untracked file called ‘hello-world.js’.
We then run:
> git add hello-world.js
This will track the files and get them ready for a commit.
Finally, we commit our changes and write a high-quality message so we can find out commit later. To do so, we run:
> git commit -m "finished hello world enterprise level application"
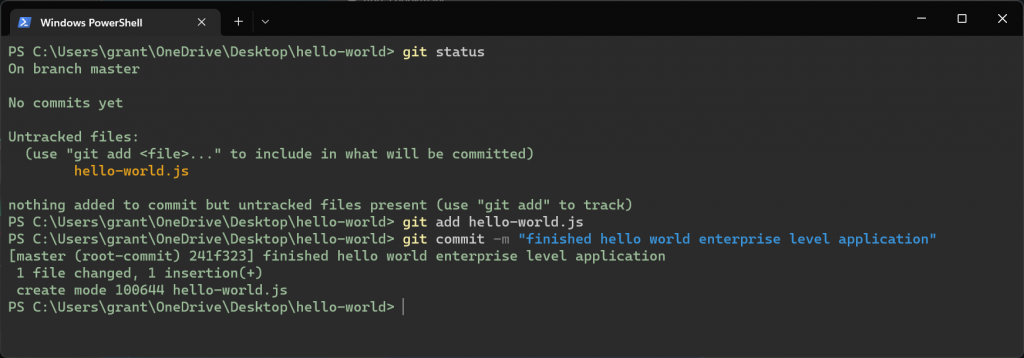
Check Git Log For Hash Number
Uh-oh! We realized our application is complete trash and don’t want a digital history of the terrible code we wrote! How do we undo this most recent commit?
Easy, all we have to do is find the hash from the commit we want to revert to and revert it.
To start, let’s run the command:
> git log --oneline
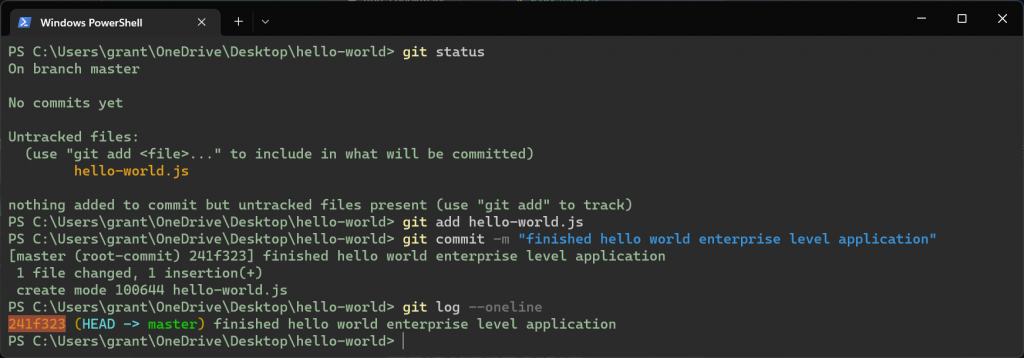
Revert Our Commit
As you can see from the above command line, we are given a list of commits we have made. Since we only made one commit, there is only one:
241f323 (HEAD -> master) finished hello world enterprise level application
The hash we are looking for is ‘241f323’. If you had multiple commits, you would need to search for the commit you want to revert. This is why messages are important!
Now let’s revert the changes by running the command:
> git revert 241f323 --no-edit
The ‘–no-edit’ portion of the command is simply used to remove the necessity to write a message. Don’t worry, this change will be obvious without your message. As we will see below.
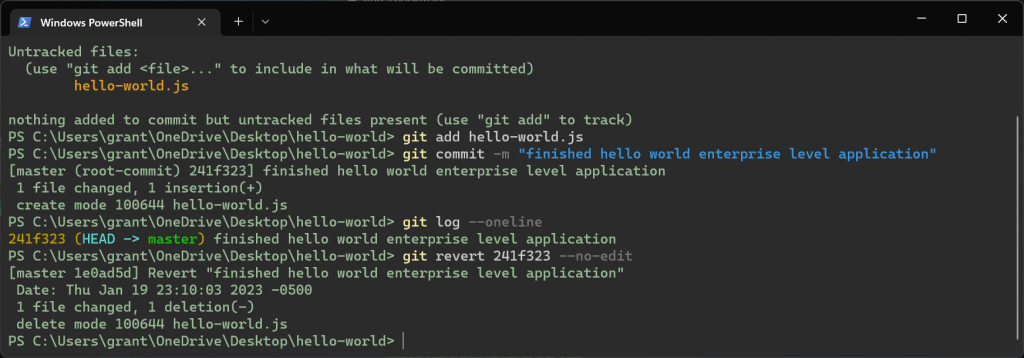
That’s it! You should have successfully reverted your changes. If you look back at your code editor, the code you wrote should be gone.
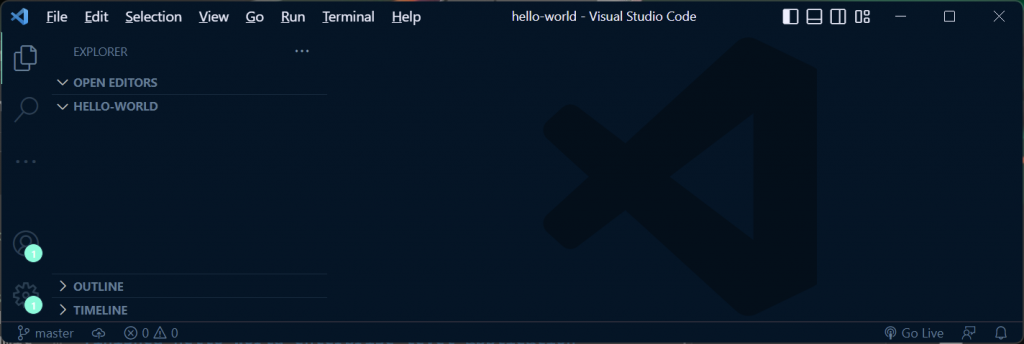
Adding A Revert Commit
Now, this little trick doesn’t exactly ‘undo’ your changes. Rather, it creates an additional commit that removes all the changes you made in the identified commit. If we run that ‘git log –oneline’ command again, it will show us there are now two commits, not zero!
One is our original commit, and the second is our ‘reverted’ commit that removed those changes.

1e0ad5d(HEAD -> master) Revert "finished hello world enterprise level application"
241f323 finished hello world enterprise level application
While you may just want to forget about that dumb mistake you made, there is a reason Git does this. If you ever want to revert back and see those original changes you made, you easily can with ‘git checkout’!
Summary: Undoing Local Commits In Git
So that’s pretty much all you need to know to undo local commits in Git! It is really as easy as running ‘git revert [hash] –no-edit’. However, you need to know the hash of the commit you want to revert. That is why we run ‘git log –oneline’.
Are you working with Git but don’t feel as comfortable with the tool as you would like to be? Check out this top-selling course on udemy and you’ll be a professional in no time.
Happy coding!